Responsibility of Laravel Controller
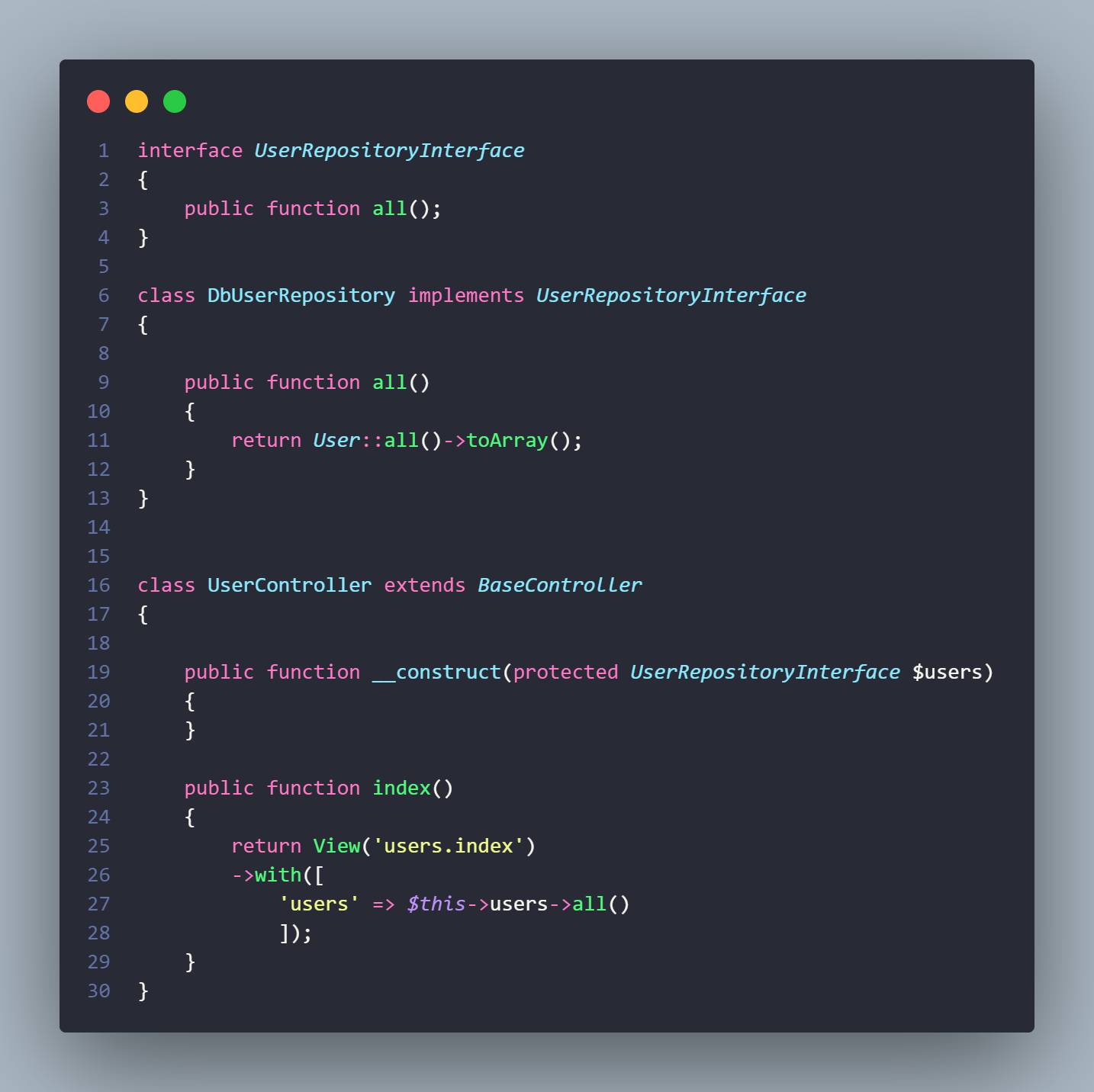
Laravel controllers are designed to be agnostic to the data source. They should not be aware of whether the data is stored in MySQL, PostgreSQL, or another database system. Instead, their responsibility is to provide a consistent interface for accessing and manipulating data, regardless of its source.
This approach has several benefits:
- Flexibility: Controllers can be easily reused with different data sources. This can be useful for developing applications that need to support multiple databases or that need to be migrated to a new database system in the future.
- Testability: Controllers can be unit tested without requiring access to a real database. This can make tests faster and more reliable.
- Maintainability: Controllers are easier to maintain because they do not need to be updated every time the data source is changed.
To achieve data source agnosticism, Laravel controllers typically use a data abstraction layer (DAL). A DAL is a layer of software that provides a consistent interface for accessing and manipulating data from different data sources. This allows controllers to interact with the data source without needing to know the specific details of the implementation.
For example, a Laravel controller might have a method called getUser()
that returns a user object from the database. The controller does not need to know whether the data is stored in MySQL, PostgreSQL, or another database system. Instead, it simply calls the getUser()
method on the DAL. The DAL then handles the details of retrieving the user data from the specific database system.
Overall, Laravel controllers are designed to be flexible, testable, and maintainable. By being agnostic to the data source, controllers can be easily reused with different data sources and can be unit tested without requiring access to a real database. This makes controllers easier to develop and maintain.
Here is a simple example of a Laravel controller that is agnostic to the data source:
userRepository = $userRepository;
}
public function getUser($id)
{
return $this->userRepository->find($id);
}
public function getAllUsers()
{
return $this->userRepository->all();
}
}
This controller has two methods: getUser() and getAllUsers(). The getUser() method takes an ID as input and returns the corresponding user object. The getAllUsers() method returns a collection of all users.
Both of these methods use the UserRepository class to access the user data. The UserRepository class is a data abstraction layer that provides a consistent interface for accessing and manipulating user data, regardless of the underlying data source.
For example, the UserRepository class might have a find() method that retrieves a user object from the database by its ID. The UserRepository class might also have an all() method that retrieves a collection of all users from the database.
The UserController class does not need to know the specific details of how the UserRepository class retrieves the user data. It simply calls the find() or all() method on the UserRepository class, and the UserRepository class handles the details of retrieving the data from the underlying data source.
This approach makes the UserController class more flexible, testable, and maintainable. The controller can be easily reused with different data sources, and it can be unit tested without requiring access to a real database.
Good, this will help new developers a lot, Nice work keep it up!
Thank you! We appreciate your positive feedback and support. We’ll continue to work hard to assist new developers and improve our offerings.